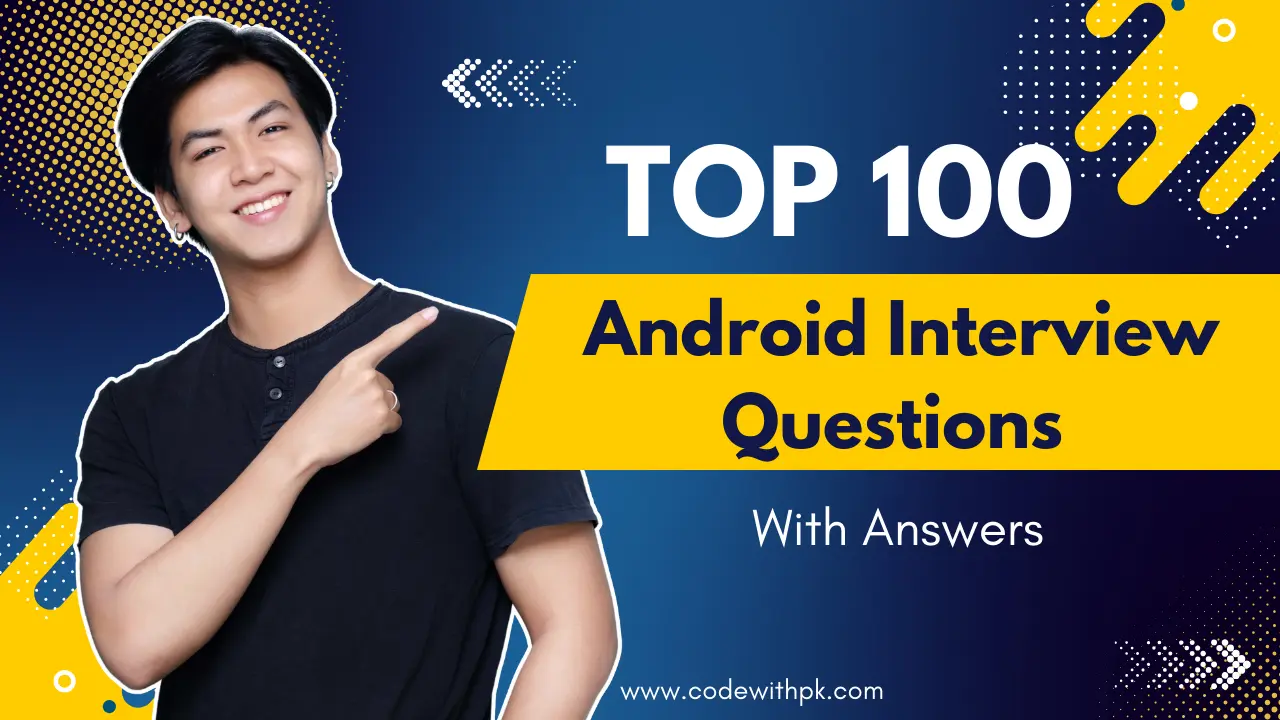
Here’s a comprehensive list of Android interview questions with short answers, perfect for preparing for interviews! π
1οΈβ£ Basic Android Questions π±
Q1: What is Android?
π Answer: Android is an open-source operating system developed by Google for mobile devices like smartphones and tablets.
Q2: What is the Android Architecture?
π Answer: It includes the Linux Kernel, Libraries, Android Runtime, Application Framework, and Applications.
Q3: What is an Activity?
π Answer: An Activity represents a single screen with a user interface in an Android app.
Q4: What is an Intent?
π Answer: An Intent is used to start activities, services, or broadcast receivers.
Q5: What is a Service?
π Answer: A Service is a component used to perform background tasks without user interaction.
Q6: What is a Content Provider?
π Answer: A Content Provider manages shared app data, such as accessing contacts or media files.
Q7: What is a Broadcast Receiver?
π Answer: It listens for system or app-wide broadcast messages like battery low or SMS received.
Q8: What is a Fragment?
π Answer: A Fragment represents a portion of UI within an Activity, making it reusable.
Q9: What is a View in Android?
π Answer: A View is a building block of UI components like buttons, text fields, or layouts.
Q10: What is an Android Manifest File?
π Answer: The AndroidManifest.xml file declares app components, permissions, and app metadata.
2οΈβ£ Activity Lifecycle Questions π
Q11: What are the states of an Activity lifecycle?
π Answer: onCreate(), onStart(), onResume(), onPause(), onStop(), onDestroy().
Q12: What is onSaveInstanceState()?
π Answer: It saves temporary data when an Activity is about to be destroyed.
Q13: What is onRestoreInstanceState()?
π Answer: It restores saved data during configuration changes or app restarts.
Q14: What is the difference between onPause() and onStop()?
π Answer: onPause() is called when the Activity is partially visible, while onStop() is called when it is completely hidden.
Q15: What is onBackPressed() used for?
π Answer: It handles the back button press action.
Q16: What is the difference between onCreate() and onStart()?
π Answer: onCreate() initializes the Activity, while onStart() makes it visible to the user.
Q17: What is the purpose of the onDestroy() method?
π Answer: It is called when the Activity is destroyed to release resources.
Q18: What is the purpose of finish()?
π Answer: It ends the Activity and removes it from the stack.
Q19: What is a configuration change?
π Answer: A change in device configuration, like screen rotation or language change, that can restart the Activity.
Q20: How do you handle configuration changes?
π Answer: Use android:configChanges
in the Manifest or override onConfigurationChanged()
.
3οΈβ£ Android Components Questions π¦
Q21: What are the main components of an Android app?
π Answer: Activities, Services, Broadcast Receivers, and Content Providers.
Q22: What is an Application class?
π Answer: It is a base class that contains global application state and can be overridden.
Q23: What are Resources in Android?
π Answer: Resources are external files such as strings, layouts, and images used in an app.
Q24: What is a Drawable?
π Answer: A Drawable is a resource that defines graphics, such as bitmaps or shapes, for UI elements.
Q25: What is an APK file?
π Answer: APK stands for Android Package and is the file format used for Android app distribution.
Q26: What is an AAR file?
π Answer: An Android Archive (AAR) is a library project package that includes resources, code, and assets.
Q27: What is Gradle?
π Answer: Gradle is a build tool used to compile, package, and manage dependencies in Android projects.
Q28: What is a Content Resolver?
π Answer: It provides access to data from Content Providers.
Q29: What is the Dalvik Virtual Machine (DVM)?
π Answer: DVM is the original virtual machine for running Android apps, replaced by ART in newer versions.
Q30: What is ART?
π Answer: Android Runtime (ART) is the modern runtime environment for Android apps, replacing DVM.
4οΈβ£ Advanced Android Questions π
Q31: What is ProGuard?
π Answer: ProGuard is a tool used to shrink, optimize, and obfuscate the code to make it more secure.
Q32: What is Multidex in Android?
π Answer: Multidex allows apps with more than 64K methods to be split across multiple DEX files.
Q33: What is Android Jetpack?
π Answer: A suite of libraries, tools, and guidance provided by Google to help build robust Android apps.
Q34: What is Data Binding?
π Answer: A library that binds UI components to data sources in XML layouts.
Q35: What is ViewModel in Android?
π Answer: A component that stores UI-related data and survives configuration changes.
Q36: What is LiveData?
π Answer: LiveData is an observable data holder class that updates the UI automatically when data changes.
Q37: What is WorkManager?
π Answer: WorkManager is a library used for managing deferrable and guaranteed background tasks.
Q38: What is Room Database?
π Answer: A persistence library that provides an abstraction layer over SQLite.
Q39: What is Coroutine in Kotlin?
π Answer: Coroutines are lightweight threads for asynchronous programming in Kotlin.
Q40: What is Dependency Injection (DI)?
π Answer: A technique for providing objects that an app’s components require, often implemented using Dagger or Hilt.
5οΈβ£ Layout and UI Questions π¨
Q41: What is a ConstraintLayout?
π Answer: A flexible layout that allows you to position and size widgets in a relative way.
Q42: What is the difference between LinearLayout and RelativeLayout?
π Answer: LinearLayout arranges views linearly, while RelativeLayout positions views relative to each other or the parent.
Q43: What is RecyclerView?
π Answer: A modern and efficient version of ListView for displaying large datasets.
Q44: What is the ViewHolder pattern?
π Answer: A design pattern used to improve performance by reusing views in RecyclerView.
Q45: What is View Binding?
π Answer: A feature that generates a binding class for each XML layout file.
Q46: What is a CardView?
π Answer: A view that provides a rounded corner background with shadows, commonly used in Material Design.
Q47: What is Material Design?
π Answer: A design system by Google for creating visually appealing and consistent UI across apps.
Q48: What is the CoordinatorLayout?
π Answer: A super-powered FrameLayout for handling transitions and animations between child views.
Q49: What is a NestedScrollView?
π Answer: A scroll view that supports nested scrolling in Android.
Q50: How do you create custom views in Android?
π Answer: Extend the View
class and override its methods like onDraw()
and onMeasure()
.
6οΈβ£ Android Performance Questions β‘
Q51: What is ANR?
π Answer: ANR (Application Not Responding) occurs when the main thread is blocked for too long.
Q52: How do you avoid ANR?
π Answer: Perform long-running operations on background threads using AsyncTask, Handlers, or Coroutines.
Q53: What is memory leak in Android?
π Answer: It occurs when an object is no longer needed but still referenced, preventing garbage collection.
Q54: How do you prevent memory leaks?
π Answer: Use weak references, avoid static contexts, and unregister listeners.
Q55: What is StrictMode?
π Answer: A tool to detect and fix performance issues like disk and network access on the main thread.
Q56: What is ProGuard optimization?
π Answer: It reduces app size and makes reverse engineering more difficult.
Q57: What is the difference between Dalvik and ART?
π Answer: ART has ahead-of-time compilation, leading to faster app performance compared to Dalvik.
Q58: What is the use of TraceView?
π Answer: A tool to profile app performance and identify bottlenecks.
Q59: What is overdraw in Android?
π Answer: Overdraw occurs when a pixel is drawn multiple times in the same frame, reducing performance.
Q60: How do you optimize the app size?
π Answer: Use ProGuard, remove unused resources, and enable APK splits for different screen densities.
7οΈβ£ Android Security Questions π
Q61: What is Android Keystore System?
π Answer: A secure system to store cryptographic keys for encryption and decryption.
Q62: How do you secure sensitive data in Android?
π Answer: Use encrypted shared preferences, Android Keystore, or SQLCipher for database encryption.
Q63: What is ProGuard in terms of security?
π Answer: ProGuard helps obfuscate code to prevent reverse engineering.
Q64: What is HTTPS in Android?
π Answer: A secure protocol for transferring data between app and server using SSL/TLS encryption.
Q65: What is the purpose of Network Security Configuration?
π Answer: It allows developers to configure security settings like certificate pinning in XML.
Q66: What is certificate pinning?
π Answer: A technique to protect against man-in-the-middle attacks by restricting trusted certificates.
Q67: How do you prevent SQL Injection in Android?
π Answer: Use parameterized queries or ORM libraries like Room.
Q68: What is the role of permissions in Android security?
π Answer: Permissions control access to sensitive data and system features.
Q69: What are Runtime Permissions?
π Answer: Permissions requested at runtime to provide more control over user data access.
Q70: How do you secure API keys in Android?
π Answer: Store them in a secure backend or encrypted in native libraries using NDK.
8οΈβ£ Android Networking Questions π
Q71: What is Retrofit?
π Answer: A popular library for making HTTP calls and parsing responses in Android.
Q72: What is OkHttp?
π Answer: A powerful HTTP client library used for efficient networking.
Q73: What is Volley?
π Answer: A library for handling network requests and image loading in Android.
Q74: What is WebView?
π Answer: A component that displays web content within an Android app.
Q75: What is the difference between REST and SOAP?
π Answer: REST is lightweight and JSON-based, while SOAP is protocol-based and XML-heavy.
Q76: What is a JSON in Android?
π Answer: JSON (JavaScript Object Notation) is a lightweight format for data exchange.
Q77: How do you parse JSON in Android?
π Answer: Use libraries like Gson, Moshi, or Kotlin serialization.
Q78: What is a NetworkOnMainThreadException?
π Answer: It occurs when networking operations are performed on the main thread.
Q79: How do you upload files in Android?
π Answer: Use libraries like Retrofit or HTTP client libraries to handle file uploads.
Q80: What is Firebase Realtime Database?
π Answer: A cloud-hosted NoSQL database that synchronizes data in real-time across clients.
9οΈβ£ Android Testing Questions π§ͺ
Q81: What is Unit Testing?
π Answer: A type of testing focused on individual components like functions or classes.
Q82: What is Instrumentation Testing?
π Answer: Tests that run on an Android device or emulator, interacting with UI components.
Q83: What is Espresso?
π Answer: A testing framework for writing UI tests in Android.
Q84: What is Robolectric?
π Answer: A framework that runs Android tests on a JVM without an emulator.
Q85: What is Monkey Testing?
π Answer: Automated random testing to check app stability under unpredictable inputs.
Q86: What is UI Automator?
π Answer: A tool for testing interactions with apps outside your app’s scope.
Q87: What is Mockito?
π Answer: A library used for mocking dependencies in Android tests.
Q88: How do you test database operations?
π Answer: Use in-memory databases like Roomβs TestDatabase for isolated testing.
Q89: What is Code Coverage?
π Answer: A metric that measures how much of your code is executed during tests.
Q90: How do you write effective test cases?
π Answer: Follow practices like defining inputs, expected outputs, and edge cases.
π Publishing & Maintenance Questions π€
Q91: What is an APK?
π Answer: Android Package (APK) is the file format used to distribute and install Android apps.
Q92: What is the Play Store Console?
π Answer: A platform for publishing and managing Android apps on Google Play Store.
Q93: What is App Bundle?
π Answer: A new publishing format that optimizes APK delivery to devices.
Q94: What is versionCode and versionName?
π Answer: versionCode is an integer for app updates, and versionName is a user-friendly version string.
Q95: How do you handle app crashes?
π Answer: Use tools like Firebase Crashlytics to analyze crash reports and fix issues.
Q96: What is A/B Testing?
π Answer: A technique for testing different versions of app features to see which performs better.
Q97: What is the importance of user feedback?
π Answer: Feedback helps improve the appβs features, usability, and overall performance.
Q98: How do you deprecate old features?
π Answer: Gradually replace them with new features while maintaining compatibility.
Q99: What are app analytics?
π Answer: Tools like Google Analytics and Firebase to track user behavior and app performance.
Q100: What is App Localization?
π Answer: Adapting the appβs language, currency, and content for different regions.
π Thanks for reading! βοΈ Letβs keep coding and building amazing apps! π π