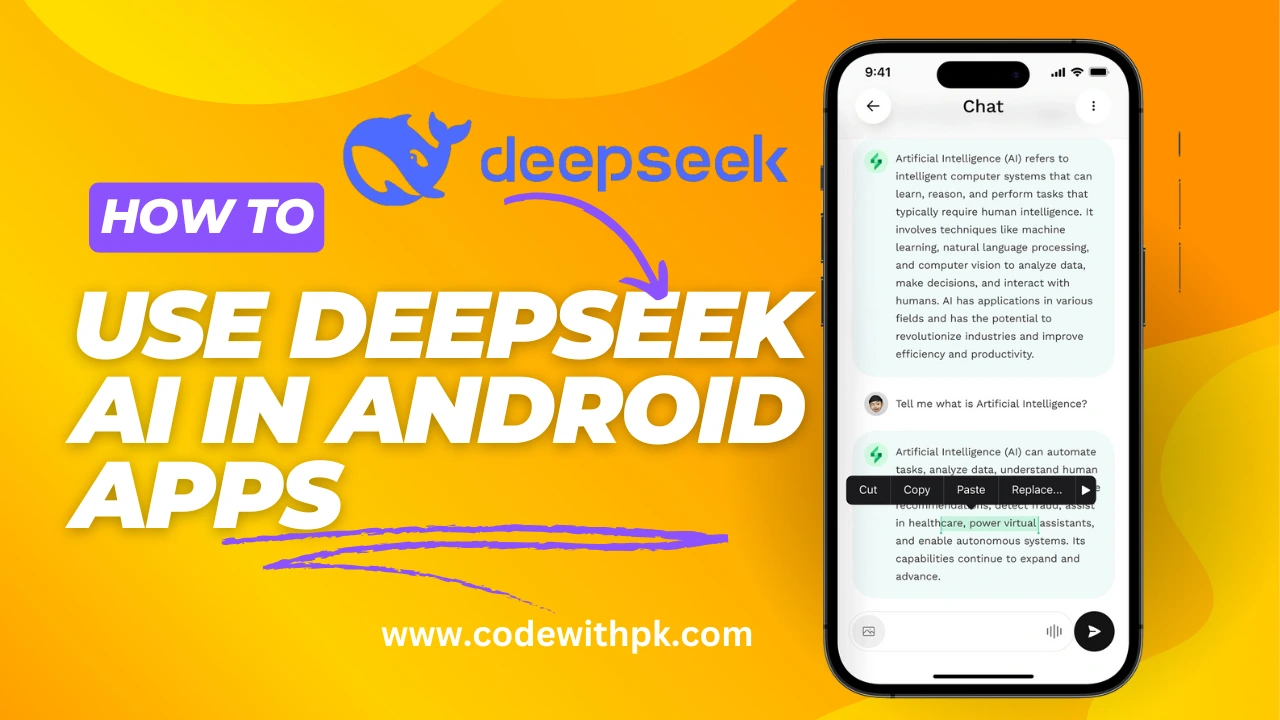
DeepSeek is a powerful deep-learning model that can be integrated into Android apps for tasks like conversational AI. In this article, youβll learn how to download the DeepSeek model from HuggingFace, convert it to TensorFlow Lite (TFLite), and create a ChatGPT-like interface using Kotlin and Jetpack Compose.
1. Download the DeepSeek Model from HuggingFace π₯
- Visit the HuggingFace repository where the DeepSeek model is hosted.
- URL: HuggingFace
- Use the
transformers
library to download the model programmatically.
Python Code to Download the Model
from transformers import AutoTokenizer, AutoModel
# Specify the model name
model_name = "deepseek/deepseek-model"
# Download the tokenizer and model
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
# Save the model locally
model.save_pretrained("./deepseek_model")
tokenizer.save_pretrained("./deepseek_model")
2. Convert the Model to ONNX π
The ONNX format is a standard for AI models, enabling interoperability between frameworks.
Steps to Convert to ONNX
- Install the required libraries:
pip install onnx onnxruntime transformers onnxruntime-tools
- Use the following script to convert the DeepSeek model to ONNX:
from transformers import AutoTokenizer, AutoModel from transformers.onnx import export model_name = "./deepseek_model" # Local path to the saved model export_path = "./deepseek_model.onnx" # Convert to ONNX export(model_name, export_path, opset=11) print("Model converted to ONNX format!")
3. Convert ONNX to TensorFlow π‘
TensorFlow is required for further optimization and compatibility with Android.
Steps to Convert to TensorFlow
- Install ONNX to TensorFlow converter:
pip install onnx-tf
- Convert the ONNX model:
from onnx_tf.backend import prepare import onnx # Load the ONNX model onnx_model = onnx.load("./deepseek_model.onnx") # Convert to TensorFlow model tf_rep = prepare(onnx_model) tf_rep.export_graph("./deepseek_model_tf") print("Model converted to TensorFlow format!")
4. Convert TensorFlow to TensorFlow Lite β‘
TFLite is optimized for mobile devices and is essential for deploying the model on Android.
Steps to Convert to TFLite
- Use the TensorFlow Lite Converter:
import tensorflow as tf # Convert to TFLite converter = tf.lite.TFLiteConverter.from_saved_model("./deepseek_model_tf") converter.optimizations = [tf.lite.Optimize.DEFAULT] tflite_model = converter.convert() # Save the TFLite model with open("deepseek_model.tflite", "wb") as f: f.write(tflite_model) print("Model converted to TFLite format!")
- Test the TFLite model on your local machine before deploying it to Android.
5. Integrate the TFLite Model into an Android App π±
Now, letβs use the TFLite model in an Android app to create a ChatGPT-like interface.
Steps to Integrate the Model
- Add TensorFlow Lite Dependencies: Add the following to your
build.gradle
file:implementation 'org.tensorflow:tensorflow-lite:2.12.0' implementation 'org.tensorflow:tensorflow-lite-support:0.4.0'
- Add the TFLite Model: Place the
deepseek_model.tflite
file in theassets
directory. - Build the Kotlin Code: Below is the complete Kotlin code for a Jetpack Compose-based chat interface:
Kotlin Code for Android App
package com.example.deepseekchat
import android.content.res.AssetFileDescriptor
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.text.BasicTextField
import androidx.compose.material3.*
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import kotlinx.coroutines.Dispatchers
import kotlinx.coroutines.withContext
import org.tensorflow.lite.Interpreter
import java.io.FileInputStream
import java.nio.MappedByteBuffer
import java.nio.channels.FileChannel
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
DeepSeekChatApp()
}
}
private fun loadModelFile(): MappedByteBuffer {
val fileDescriptor: AssetFileDescriptor = assets.openFd("deepseek_model.tflite")
val inputStream = FileInputStream(fileDescriptor.fileDescriptor)
val fileChannel: FileChannel = inputStream.channel
return fileChannel.map(FileChannel.MapMode.READ_ONLY, fileDescriptor.startOffset, fileDescriptor.declaredLength)
}
private suspend fun runModel(inputText: String): String = withContext(Dispatchers.Default) {
val interpreter = Interpreter(loadModelFile())
// Preprocess input and run the model here (not shown for simplicity)
val outputText = "Model response for: $inputText" // Replace with real model output
interpreter.close()
outputText
}
@Composable
fun DeepSeekChatApp() {
var query by remember { mutableStateOf("") }
var response by remember { mutableStateOf("Hi! How can I help you?") }
Column(
Modifier.fillMaxSize().padding(16.dp),
verticalArrangement = Arrangement.SpaceBetween
) {
Text("DeepSeek Chat", style = MaterialTheme.typography.h5)
Spacer(modifier = Modifier.height(16.dp))
Box(
Modifier.weight(1f).padding(8.dp)
) {
Text(response)
}
Spacer(modifier = Modifier.height(16.dp))
Row(Modifier.fillMaxWidth()) {
BasicTextField(
value = query,
onValueChange = { query = it },
Modifier.weight(1f).padding(8.dp)
)
Button(onClick = {
response = runModel(query)
}) {
Text("Send")
}
}
}
}
}
6. Flow Diagram π οΈ
Hereβs the flow of the entire process:
Download Model (HuggingFace)
β
Convert to ONNX
β
Convert to TensorFlow
β
Optimize to TFLite
β
Integrate into Android App
β
Run the Model for Chat Queries
7. Run the App and Test π
- Build and run the app on an Android device.
- Enter a query in the chat box and press “Send.”
- The app will process your input using the TFLite model and display the model’s response.
Key Features of the App π
- Minimalistic and user-friendly chat interface.
- Real-time inference using TensorFlow Lite.
- Fully optimized for mobile devices.
Conclusion π
By following this guide, youβve learned how to use the DeepSeek model in an Android app, from downloading the model to deploying it with a ChatGPT-like interface. This process demonstrates how to leverage the power of AI on mobile devices, making your apps smarter and more interactive.