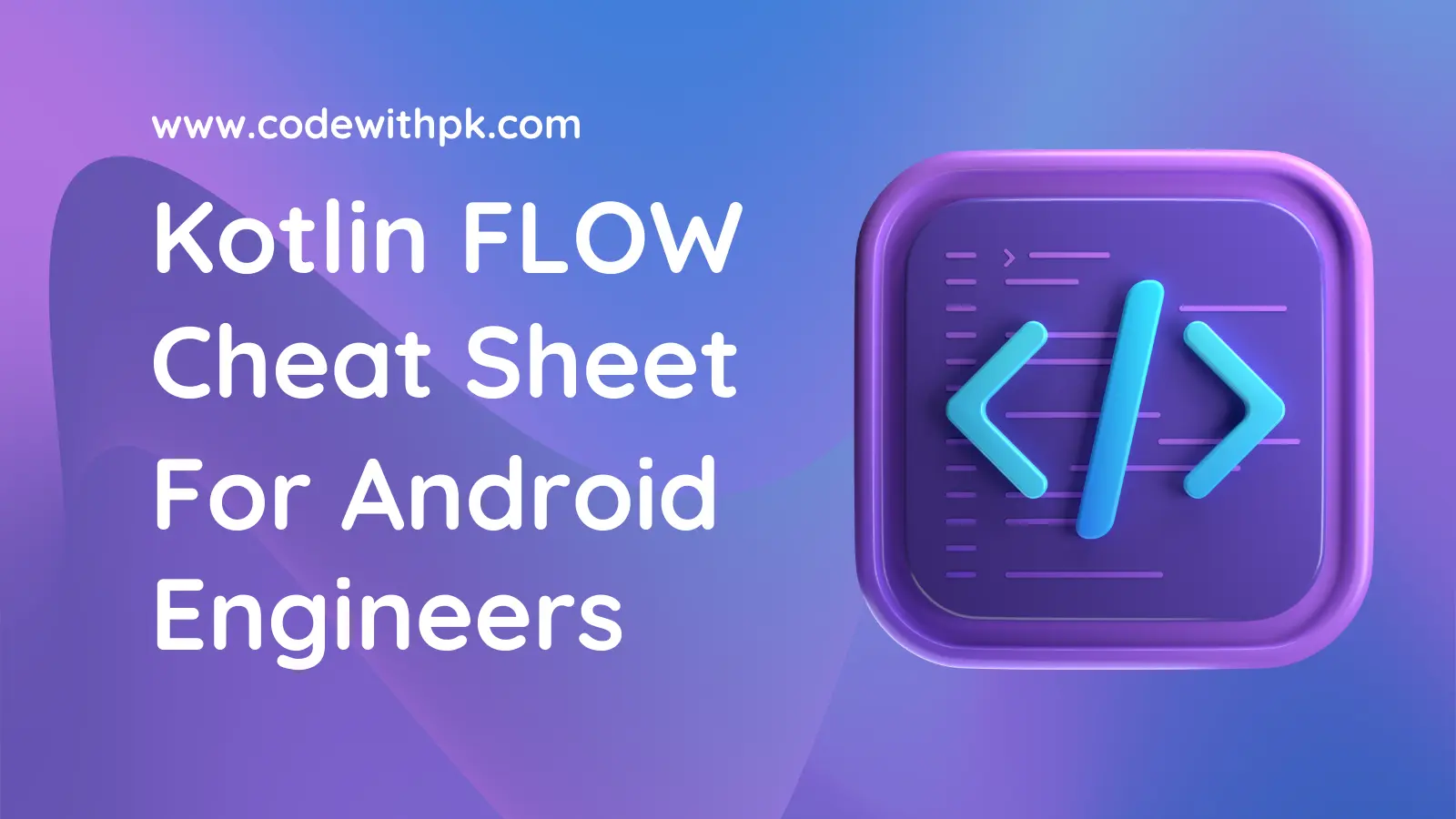
Are you an Android Engineer diving deep into Kotlin Flows? Do you get stuck on concepts like channels, zip vs. combine, or the difference between SharedFlow and StateFlow? Youβre in the right place! This cheat sheet will simplify advanced Flow concepts, helping you master them in no time.
π§ What is Kotlin Flow?
Kotlin Flow is a powerful asynchronous stream library that supports sequential, concurrent, and reactive programming in Android. It’s built on coroutines and provides a modern replacement for RxJava.
Basic Traits:
- Lazy: Emits values only when collected.
- Backpressure-free.
- Supports transformations, operators, and error handling.
π§οΈ Cold Streams vs. π₯ Hot Streams
π§οΈ Cold Streams
- Examples:
Flow
,Sequence
. - Key Traits:
- Values are emitted only when collected.
- Each subscriber gets its own independent sequence of values.
π₯ Hot Streams
- Examples:
Channel
,SharedFlow
,StateFlow
. - Key Traits:
- Values are emitted immediately, even without collectors.
- Shared state: Subscribers receive the same data.
π’ Channels: The Backbone of Coroutines
π What Are Channels?
Channels are hot streams used for communication between coroutines. They ensure thread safety without explicit locks.
π§ How Do They Work?
- Channels work like queues.
- FIFO (First-In-First-Out) behavior ensures fairness.
- Each value is received only once by a single consumer.
π Example: Channel Basics
val channel = Channel<String>()
// Coroutine 1: Sender
launch {
channel.send("Hello")
channel.send("World")
channel.close() // Always close after sending all values
}
// Coroutine 2: Receiver
launch {
for (msg in channel) {
println(msg) // Output: Hello, World
}
}
π Channel Buffer Types
Channels come with different buffer options:
Channel.UNLIMITED
: Infinite buffer size.Channel.RENDEZVOUS
: No buffer, sends only when received.Channel.BUFFERED
: Default buffer size (64 items).Channel.CONFLATED
: Keeps only the latest value.Channel(n)
: Custom buffer size.
π Combine, Merge, and Zip: Whatβs the Difference?
βοΈ Merge
- Combines multiple flows into a single flow.
- Emits values as soon as theyβre available, without waiting for others.
Example:
val flow1 = flowOf(1, 2)
val flow2 = flowOf(3, 4)
merge(flow1, flow2).collect { println(it) }
// Output: 1, 3, 2, 4
βοΈ Zip
- Combines flows pairwise.
- Waits for all flows to emit before combining values.
Example:
val flow1 = flowOf(1, 2)
val flow2 = flowOf(3, 4)
flow1.zip(flow2) { a, b -> a + b }.collect { println(it) }
// Output: 4, 6
πΏ Combine
- Combines flows into a new flow.
- Emits a new value whenever any flow emits.
Example:
val flow1 = flowOf(1, 2)
val flow2 = flowOf(3, 4)
combine(flow1, flow2) { a, b -> a + b }.collect { println(it) }
// Output: 4, 5, 6
π Transforming Flows: FlatMapConcat vs. FlatMapMerge vs. FlatMapLatest
π flatMapConcat
- Processes each flow sequentially.
- Waits for one flow to complete before starting the next.
Example:
val flow = flowOf(1, 2)
flow.flatMapConcat { value ->
flowOf(value * 2)
}.collect { println(it) }
// Output: 2, 4
π flatMapMerge
- Processes flows concurrently.
- Results are emitted as soon as theyβre ready.
Example:
val flow = flowOf(1, 2)
flow.flatMapMerge { value ->
flowOf(value * 2)
}.collect { println(it) }
// Output: 2, 4
β³ flatMapLatest
- Cancels the previous flow whenever a new value is emitted.
Example:
val flow = flowOf(1, 2)
flow.flatMapLatest { value ->
flowOf(value * 2)
}.collect { println(it) }
// Output: 2, 4
π StateFlow vs. SharedFlow
π‘ StateFlow
- A hot flow that always holds the latest value.
- Perfect for state management.
Example:
val stateFlow = MutableStateFlow("Initial")
stateFlow.value = "Updated"
stateFlow.collect { println(it) }
// Output: Updated
π SharedFlow
- A hot flow that can emit multiple values to subscribers.
- Great for events like navigation.
Example:
val sharedFlow = MutableSharedFlow<String>()
sharedFlow.emit("Navigate")
sharedFlow.collect { println(it) }
// Output: Navigate
π Advanced Operators
π€Ώ fold vs. scan
- fold: Produces a final result (e.g., sum of all values).
- scan: Emits intermediate results.
Example:
val flow = flowOf(1, 2, 3)
flow.scan(0) { acc, value -> acc + value }.collect { println(it) }
// Output: 0, 1, 3, 6
π Pro Tips for Mastering Flows
- Use StateFlow for UI state and SharedFlow for one-time events.
- Prefer flatMapMerge for faster processing, but use flatMapConcat when order matters.
- Experiment with buffering to handle backpressure.
- Use catch and retry for robust error handling.
πͺ With this cheat sheet, youβre well on your way to mastering Kotlin Flows. Start applying these concepts to your Android projects today and unlock their full potential! π
Happy Coding! π
π Join Professional Developer Group
Building community of developers. Join Now! Be part of this thriving group to level up your career.