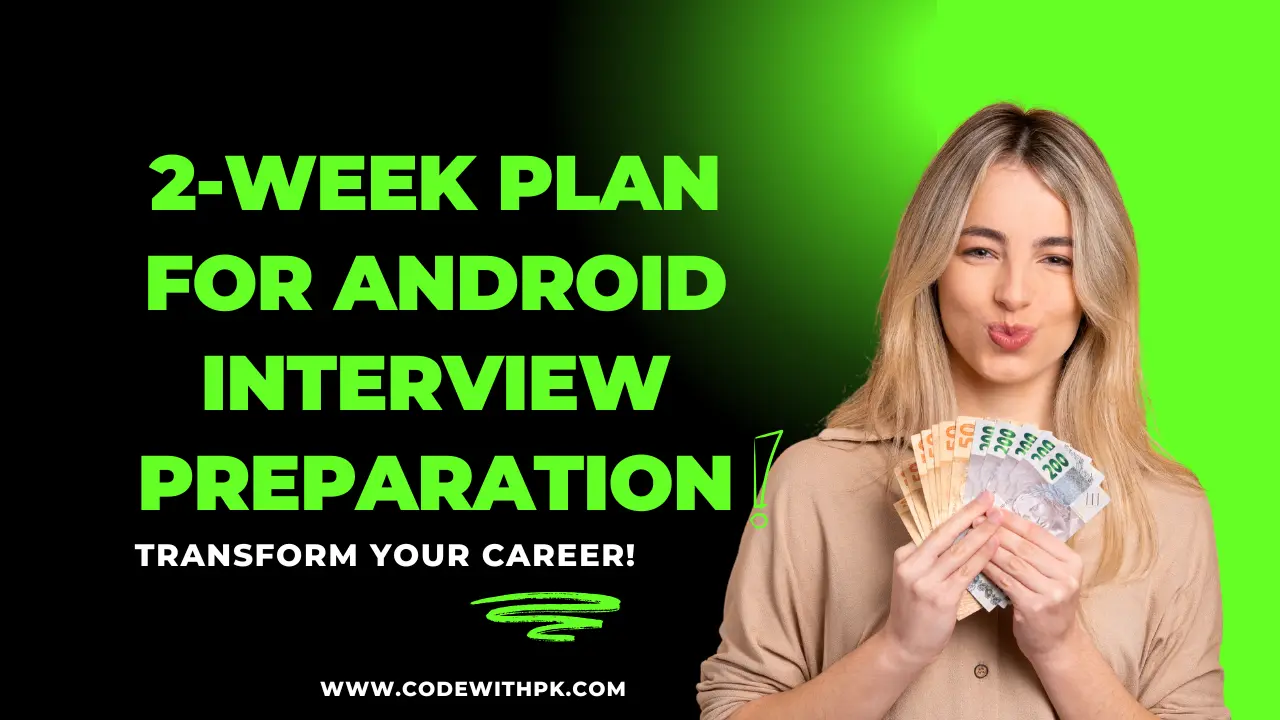
Preparing for an Android interview with 3+ years of experience requires focusing on Kotlin-first Android, modern technologies, and DSA fundamentals. Here’s a 14-day roadmap to help you ace your interview. It assumes you can dedicate 6-8 hours daily.
Week 1: Core Android and DSA
Day 1: Android Fundamentals π±
Key Topics:
- Core Android Concepts:
- Activity & Fragment Lifecycle π
- Context types: Application, Activity, Service
- Intents: Explicit, Implicit, PendingIntents
- Services: Foreground, Background, Bound Services
- Jetpack Components:
- ViewModel, LiveData, Navigation, WorkManager
- Lifecycle-aware best practices
DSA Focus: Arrays π§©
- Problems to Solve:
- Kadaneβs Algorithm
- Subarray Sum Equals K
- Sliding Window problems
- Platforms: LeetCode, HackerRank
Day 2: Modern Android Development π
Key Topics:
- Jetpack Compose Basics:
- Composable functions
- State management
- Build a basic Compose UI (e.g., login screen)
- Comparison:
- Jetpack Compose vs XML
- Jetpack Compose vs Flutter
DSA Focus: Strings π€
- Problems to Solve:
- Longest Palindromic Substring
- Group Anagrams
- String Compression
Day 3: Dependency Injection & Architecture π
Key Topics:
- Dependency Injection (DI):
- Dagger-Hilt basics
- Scopes: Singleton, ViewModelScope
- Architectural Patterns:
- MVVM vs. MVC vs. MVP
- Build a small MVVM-based app
DSA Focus: Linked Lists π
- Problems to Solve:
- Reverse a Linked List
- Detect a Cycle
- Merge Two Sorted Lists
Day 4: Multi-threading and Coroutines βοΈ
Key Topics:
- Concurrency in Android:
- Threads, Handlers, Loopers
- Kotlin Coroutines: Launch, Async, Scope
- Implementation:
- Fetch API data using coroutines
DSA Focus: Stacks & Queues π
- Problems to Solve:
- Valid Parentheses
- Min Stack
- Circular Queue
Day 5: Networking & APIs π
Key Topics:
- Networking:
- Retrofit setup with Coroutines
- API error handling with sealed classes
- REST vs GraphQL
- Implement a small REST API using Ktor
DSA Focus: Binary Trees π³
- Problems to Solve:
- Inorder, Preorder, Postorder traversal
- Max Depth of a Binary Tree
Day 6: Storage & Jetpack π
Key Topics:
- Room Database:
- Entity, DAO, Relationships
- DataStore vs SharedPreferences
DSA Focus: Binary Search Trees π²
- Problems to Solve:
- Search in a BST
- Validate BST
- Lowest Common Ancestor
Day 7: Revision & Mock Interviews π―
Key Activities:
- Solve a design problem: Create a Notes App with offline sync
- Revise Arrays, Strings, Linked Lists, Stacks, and Queues
- Solve 2-3 mixed problems
Week 2: Advanced Topics, Kotlin & System Design
Day 8: Kotlin Advanced Features π οΈ
Key Topics:
- Advanced Kotlin:
- Inline and higher-order functions
- Null safety (
let
,apply
,also
) - Delegates and extension functions
DSA Focus: Recursion π
- Problems to Solve:
- Factorial, Fibonacci
- Subsets generation
Day 9: System Design Basics ποΈ
Key Topics:
- System Design Concepts:
- Load Balancers, Caching, Sharding
- API Rate Limiting and CDNs
- Design Exercise:
- Build a design for apps like Truecaller or Ola
DSA Focus: Dynamic Programming π²
- Problems to Solve:
- Longest Increasing Subsequence
- Knapsack Problem
- House Robber
Day 10: Testing and Performance β
Key Topics:
- Testing:
- Unit Testing: JUnit, Mockito
- UI Testing: Espresso
- Performance:
- Detect and fix memory leaks (LeakCanary)
- Avoiding ANR
DSA Focus: Graphs πΊοΈ
- Problems to Solve:
- BFS, DFS
- Dijkstra’s Algorithm
Day 11: Advanced Android Features π‘
Key Topics:
- Push Notifications (FCM)
- Deep Linking and App Links
DSA Focus: Heaps & Priority Queues β«
- Problems to Solve:
- Kth Largest Element
- Merge K Sorted Lists
Day 12: Mock Interviews & Kotlin Practice π οΈ
Activities:
- Solve 3 medium DSA problems
- Build a mini-project with Room + Coroutines
Day 13: Java Refresher π
Key Topics:
- Collections Framework: HashMap, LinkedHashMap
- OOPS Basics: Polymorphism, Abstraction, Encapsulation
DSA Revision: Mixed Problems
Day 14: Final Mock Interview & Behavioral Prep π―
- Mock system design and coding rounds
- Prepare for behavioral questions
Tips for Success π‘
- Use platforms like LeetCode, HackerRank, and GFG for DSA.
- Build small projects to reinforce Android concepts.
- Focus on clarity and communication during interviews.
This plan is your path to success! Best of luck! πͺ