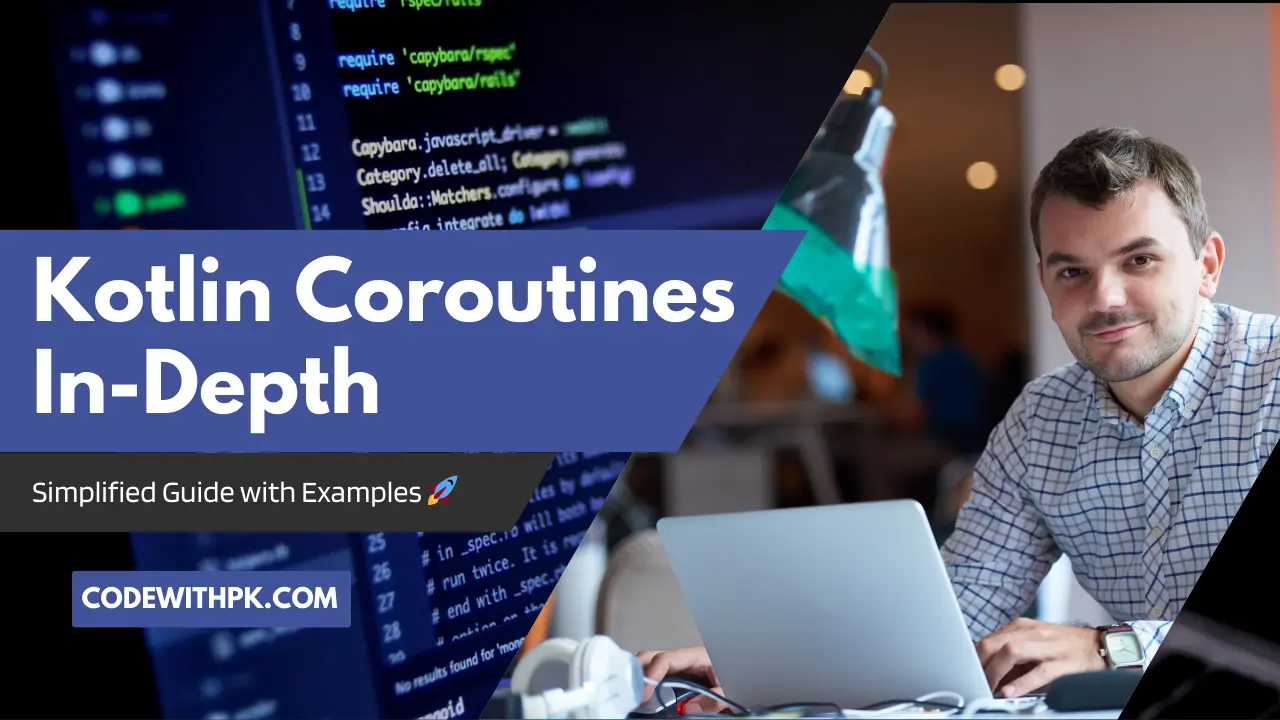
Asynchronous programming is a cornerstone of modern mobile development. It ensures apps stay responsive, smooth, and user-friendly by offloading heavy tasks from the main thread. With Kotlin Coroutines, you can handle asynchronous tasks efficiently without diving into the complexity of callbacks or threading.
In this blog, weโll explore Kotlin Coroutines in detail, breaking down concepts with simple examples and real-life use cases. Letโs dive in! ๐
What Are Coroutines? ๐ค
Coroutines are lightweight threads that enable multitasking by executing long-running tasks (e.g., API calls, database queries) in the background.
๐ก Key Features of Coroutines:
- They donโt block the main thread ๐ ๏ธ.
- They can be paused (suspended) and resumed, saving resources.
- Theyโre lightweight, allowing you to run thousands without consuming much memory.
Coroutines vs Threads ๐งต:
Feature | Coroutines | Threads |
---|---|---|
Lightweight | Yes โ , thousands in one thread | No, resource-intensive ๐ |
Non-blocking | Yes โ | Blocks thread โ |
Context Switch | Faster โก (managed by library) | Slower (OS-managed) ๐ข |
Why Use Coroutines in Android? ๐ฑ
When long-running tasks (like API calls) block the main thread, the app freezes. This leads to a poor user experience ๐ซฃ. Coroutines solve this by:
- Keeping tasks off the main thread.
- Making code cleaner and easier to read โจ.
- Reducing callback hell ๐.
Key Components of Coroutines ๐
1๏ธโฃ Coroutine Scope: Defines the lifetime of a coroutine. Common scopes in Android include:
viewModelScope
: Tied to the lifecycle of a ViewModel.lifecycleScope
: Tied to Activity or Fragment lifecycle.
2๏ธโฃ Dispatcher: Determines which thread a coroutine runs on:
Dispatchers.Main
: Main thread (UI updates ๐๏ธ).Dispatchers.IO
: Background thread (network/database work ๐).Dispatchers.Default
: CPU-intensive tasks (e.g., calculations ๐งฎ).
3๏ธโฃ Suspend Functions:
Functions marked with suspend
can pause and resume without blocking threads. Perfect for network calls and file I/O.
Getting Started with Coroutines ๐ ๏ธ
Letโs fetch weather data using coroutines:
import kotlinx.coroutines.*
// Simulate a network request
suspend fun fetchWeather(city: String): String {
delay(2000) // Simulate network delay
return "Sunny, 24ยฐC in $city"
}
fun main() = runBlocking {
println("Fetching weather data...")
val weather = fetchWeather("New York")
println("Weather data: $weather")
}
Whatโs happening here?
delay(2000)
pauses the coroutine for 2 seconds without blocking the thread.runBlocking
creates a coroutine scope for the example (used mainly in testing).
โฉ Output:
Fetching weather data...
Weather data: Sunny, 24ยฐC in New York
launch vs async: What’s the Difference? ๐คทโโ๏ธ
1๏ธโฃ launch
:
- Used for tasks that donโt return a result.
- Returns a
Job
to manage the coroutine lifecycle.
Example:
fun performBackgroundTask() {
CoroutineScope(Dispatchers.IO).launch {
delay(1000)
println("Task complete โ
")
}
}
2๏ธโฃ async
:
- Used for tasks that return a result.
- Returns a
Deferred
object, which you can use to get the result with.await()
.
Example:
suspend fun fetchData(): String {
delay(1000)
return "Data fetched successfully"
}
fun main() = runBlocking {
val deferredResult = async { fetchData() }
println("Result: ${deferredResult.await()}")
}
Real-Life Example: Fetching API Data ๐
Hereโs how to load user data and notification count simultaneously:
suspend fun fetchUserData(): String {
delay(1000) // Simulate API call
return "User: John Doe"
}
suspend fun fetchNotifications(): Int {
delay(500) // Simulate API call
return 10
}
fun main() = runBlocking {
val user = async { fetchUserData() }
val notifications = async { fetchNotifications() }
println("Dashboard: ${user.await()}, Notifications: ${notifications.await()} ๐")
}
โฉ Output:
Dashboard: User: John Doe, Notifications: 10 ๐
Error Handling in Coroutines โ ๏ธ
Use try-catch
blocks to gracefully handle errors:
suspend fun fetchData(): String {
throw Exception("Network Error ๐จ")
}
fun main() = runBlocking {
try {
val data = fetchData()
println("Data: $data")
} catch (e: Exception) {
println("Error: ${e.message}")
}
}
โฉ Output:
Error: Network Error ๐จ
Using Coroutines in Android ๐
Hereโs how to load user profiles using lifecycleScope:
class ProfileFragment : Fragment() {
override fun onViewCreated(view: View, savedInstanceState: Bundle?) {
super.onViewCreated(view, savedInstanceState)
lifecycleScope.launch(Dispatchers.IO) {
val profile = fetchUserProfile()
withContext(Dispatchers.Main) {
textViewProfile.text = profile
}
}
}
private suspend fun fetchUserProfile(): String {
delay(2000)
return "Jane Doe, Software Engineer ๐ฉโ๐ป"
}
}
Why use lifecycleScope
?
- Automatically cancels the coroutine if the Fragment is destroyed.
Advantages of Coroutines ๐
- ๐ Readable Code: Eliminates callback hell.
- โก Lightweight: Handles thousands of coroutines efficiently.
- ๐ก๏ธ Scoped Lifecycle: Reduces memory leaks in Android apps.
- โ Integrated with Jetpack: Works seamlessly with Android architecture components.
Conclusion ๐ฏ
Kotlin Coroutines simplify asynchronous programming in Android, making your apps faster, cleaner, and more efficient. Whether youโre handling API calls, file operations, or complex tasks, coroutines offer a powerful and flexible solution.
๐ Remember: Use the right scope and dispatcher, write readable code, and handle errors properly!
Thanks for reading! โ๏ธ Letโs keep coding and building amazing apps! ๐