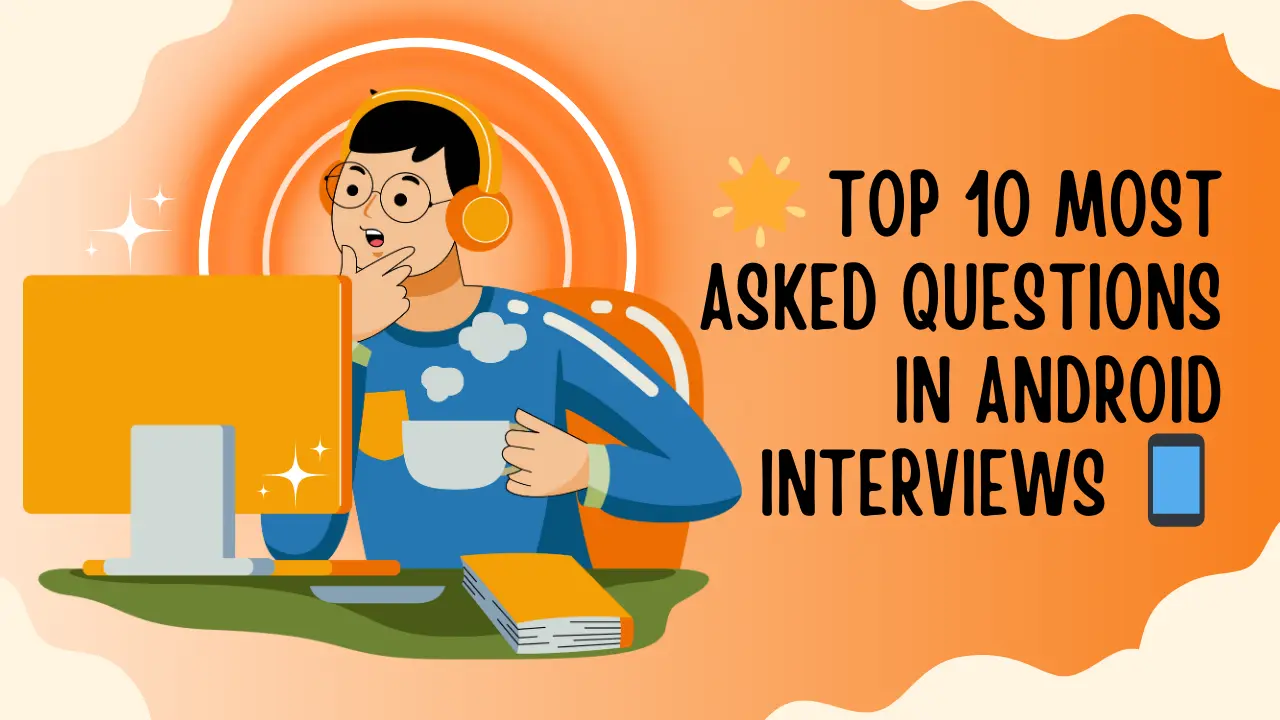
If you’re preparing for an Android interview, you’re in the right place! We’ve compiled 10 frequently asked questions with simplified explanations, real-world examples, and a touch of fun using emojis. Let’s dive in! π
1οΈβ£ Explain the Architecture Patterns Used in Android ποΈ
Android apps commonly use these patterns:
- MVVM (Model-View-ViewModel): For automatic UI updates using LiveData.
- MVP (Model-View-Presenter): Best for simpler UIs and legacy apps.
- MVI (Model-View-Intent): Ideal for unidirectional data flow.
π Example:
For a shopping cart app, use MVVM when UI needs real-time updates from the database. MVP suits simpler features like displaying a static “About Us” page.
π οΈ When to Use:
- Use MVVM for complex UIs or data-binding.
- Choose MVP for older codebases.
- Opt for MVI for apps using reactive libraries like RxJava.
2οΈβ£ How Does Android Handle Memory Management? π§
Android uses Garbage Collection (GC) to free up memory by removing unused objects. However, improper usage can cause memory leaks.
π Avoid Memory Leaks by:
- Using WeakReference for large objects.
- Avoiding long-lived references to
Context
orActivity
. - Cleaning up resources in lifecycle methods like
onDestroy()
.
π οΈ Example:
Use libraries like LeakCanary to detect memory leaks in real-time.
3οΈβ£ What is Dependency Injection (DI)? π€
Dependency Injection provides required objects (dependencies) to a class from the outside, promoting loose coupling.
Popular frameworks:
- Hilt (simpler, built on Dagger)
- Dagger (powerful, compile-time DI)
- Koin (lightweight, Kotlin DSL-based)
π― Why Use It?
- Improved Testability: Replace real dependencies with mock ones in tests.
- Loose Coupling: Change one module without affecting others.
π Example:
class UserRepository @Inject constructor(private val apiService: ApiService)
4οΈβ£ How Would You Improve App Performance? π
Boost app performance by:
- Optimizing UI rendering with tools like RecyclerView.
- Managing memory with lifecycle-aware components like
ViewModel
. - Reducing network calls by using caching with libraries like Retrofit.
π οΈ Example:
Use Glide or Picasso for efficient image loading instead of manually handling bitmaps.
5οΈβ£ What Are Coroutines? How Are They Different From Threads? π
Coroutines are lightweight and simplify asynchronous tasks, like network requests, without blocking the main thread.
π Differences:
- Coroutines are non-blocking, while threads block.
- Coroutines are managed by Kotlin; threads need OS-level management.
π Example:
launch {
val data = fetchDataFromNetwork()
updateUI(data)
}
6οΈβ£ Whatβs the Difference Between var
, val
, and const
? π
var
: Mutable variable (can change).val
: Immutable variable (cannot change).const
: Compile-time constant (immutable).
π Example:
var age = 25 // Can be reassigned
val name = "John" // Cannot be reassigned
const val PI = 3.14 // Constant at compile-time
7οΈβ£ What Are Kotlin Data Classes? ποΈ
Data classes are special classes to hold data. They automatically generate toString()
, equals()
, hashCode()
, and copy()
methods.
π Example:
data class User(val id: Int, val name: String)
val user = User(1, "Alice")
println(user) // Output: User(id=1, name=Alice)
β¨ Advantages:
- Concise and readable code.
- Built-in
copy()
for cloning objects.
8οΈβ£ What is a Sealed Class and When to Use It? π‘οΈ
A sealed class restricts subclassing to a specific set of types within the same file.
π Example:
sealed class Result {
object Success : Result()
data class Error(val message: String) : Result()
}
β¨ Use Case: For representing well-defined states like success, error, or loading in a network response.
9οΈβ£ What Are Content Providers? π
Content Providers manage access to shared data across apps. They allow one app to query or modify data in another app.
π― Use Cases:
- Sharing contacts between apps.
- Accessing media files stored by other apps.
π Example:
Use the ContentResolver
to query a provider:
val cursor = contentResolver.query(
ContactsContract.CommonDataKinds.Phone.CONTENT_URI,
null, null, null, null
)
π How Does Androidβs Jetpack Compose Simplify UI Development? π¨
Jetpack Compose is a modern toolkit for building UIs with less code and more flexibility.
π― Advantages:
- Declarative: Define what the UI should look like, and Compose handles the how.
- No XML: Say goodbye to XML layouts! Everything is Kotlin-based.
- State Management: Easier with
State
andLiveData
.
π Example:
@Composable
fun Greeting(name: String) {
Text(text = "Hello, $name!")
}
β¨ Why Use It?
Compose is perfect for modern apps that need highly interactive and adaptive UIs.
π‘ Final Tips for Your Interview
- π¬ Communicate clearly and explain your reasoning.
- π» Practice coding frequently asked problems.
- π οΈ Use real-life examples to showcase your experience.
By preparing these top 10 questions, you’ll be ready to ace your Android interview with confidence! Good luck! π