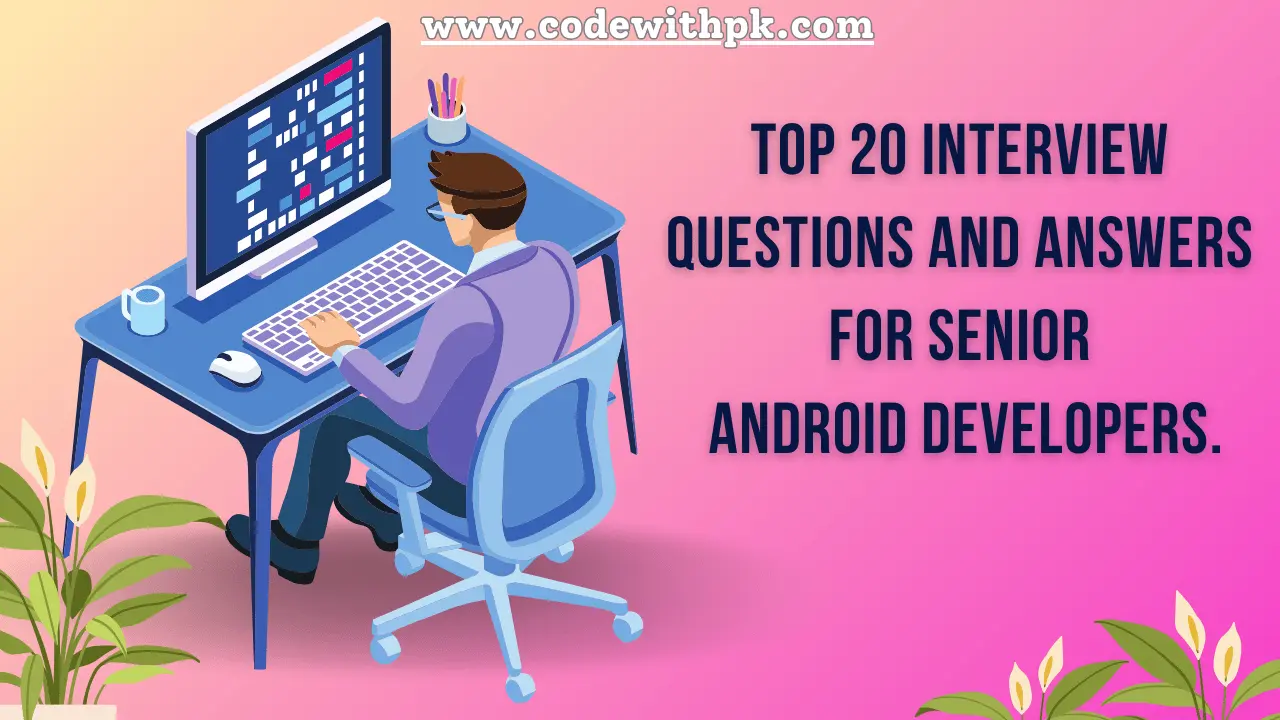
🌟 In today’s Android interviews, candidates need to showcase not just theoretical knowledge but practical expertise and problem-solving skills. Below is a list of top 20 questions frequently asked during senior Android developer interviews, along with detailed answers and examples.
Note: These questions have been previously asked in companies like Google, Meta, Amazon, and others.
1️⃣ Describe a complex app architecture you have implemented and why you chose it.
Answer:
I used the MVVM (Model-View-ViewModel) architecture in a large-scale app to ensure separation of concerns. MVVM allows the ViewModel to handle UI logic, Repositories to fetch and cache data, and LiveData to update the UI reactively.
Example in Practice:
I implemented MVVM in an e-commerce app. This made testing easier, as ViewModels were unit-tested without needing UI components. Additionally, data-binding helped keep the UI reactive and clean.
class ProductViewModel : ViewModel() {
private val repository = ProductRepository()
val products: LiveData<List<Product>> = repository.getProducts()
}
Note: Previously asked in Google interviews.
2️⃣ How do you manage dependencies in a large Android project?
Answer:
I use Hilt (Google’s Dependency Injection library) for managing dependencies. Hilt simplifies DI by providing lifecycle-aware components and reduces boilerplate code.
Example in Practice:
In a social media app, I used Hilt to inject APIs and database instances, improving modularity and testability.
@HiltAndroidApp
class MyApplication : Application()
@InstallIn(SingletonComponent::class)
@Module
class NetworkModule {
@Provides
fun provideApi(): ApiService = Retrofit.Builder().build().create(ApiService::class.java)
}
Note: Previously asked in Meta (Facebook) interviews.
3️⃣ What strategies do you use to improve the performance of an Android application?
Answer:
- Optimize layouts by reducing hierarchy depth.
- Use RecyclerView instead of ListView for efficient rendering.
- Leverage ProGuard or R8 for code shrinking.
- Avoid memory leaks by using WeakReferences and lifecycle-aware components.
- Perform background tasks using WorkManager or Coroutines.
Example in Practice:
In a delivery app, I improved performance by optimizing API calls with Retrofit and caching images with Glide. The app load time decreased by 30%.
Note: Previously asked in Flipkart interviews.
4️⃣ Explain the role of Coroutines and Flow in Kotlin for Android development.
Answer:
- Coroutines: Simplify asynchronous programming by providing a sequential syntax for background tasks, avoiding callback hell.
- Flow: A reactive stream API that handles multiple asynchronous data streams and emits values over time.
Example in Practice:
In a fintech app, I used Coroutines for fetching user data in the background and Flow to update the dashboard in real-time.
viewModelScope.launch {
val user = userRepository.getUser()
_userLiveData.postValue(user)
}
val dashboardFlow = flow {
emit(api.getDashboardData())
}
Note: Previously asked in Netflix interviews.
5️⃣ How do you approach testing in Android?
Answer:
- Unit Testing: Use JUnit and Mockito for testing business logic.
- UI Testing: Leverage Espresso for automated UI tests.
- Integration Testing: Ensure seamless interaction between app components.
- End-to-End Testing: Test complete user workflows.
Example in Practice:
I created JUnit tests to validate the payment flow in an app, ensuring edge cases like payment retries were handled properly.
@Test
fun testPaymentSuccess() {
val paymentResult = paymentProcessor.processPayment(amount = 100)
assertEquals(PaymentStatus.SUCCESS, paymentResult.status)
}
Note: Previously asked in SAP Labs interviews.
6️⃣ What is Jetpack Compose, and how does it differ from the traditional View system?
Answer:
Jetpack Compose is a declarative UI toolkit that eliminates the need for XML layouts. It enables building UI directly in Kotlin, provides state management, and updates the UI automatically through recomposition.
Example in Practice:
In an OTT app, I migrated the entire UI to Jetpack Compose, reducing boilerplate code by 50%.
@Composable
fun Greeting(name: String) {
Text(text = "Hello $name!")
}
Note: Previously asked in Amazon interviews.
7️⃣ Describe a time when you optimized an Android app for better scalability and maintainability.
Answer:
I adopted a modular architecture by splitting features into separate modules. This enabled parallel development and reduced build times. I also used ViewModels and LiveData for better state management.
Example in Practice:
In a healthcare app, I created separate modules for appointments, patient records, and notifications, making the codebase easier to manage.
Note: Previously asked in Paytm interviews.
8️⃣ How do you handle security concerns in Android applications?
Answer:
- Use EncryptedSharedPreferences for sensitive data.
- Validate user inputs to prevent SQL Injection or XSS attacks.
- Enable HTTPS with SSL pinning for secure communication.
- Obfuscate code using R8 to protect against reverse engineering.
Example in Practice:
In a banking app, I implemented EncryptedSharedPreferences to store API tokens and enabled certificate pinning for secure API calls.
Note: Previously asked in American Express interviews.
9️⃣ What is your approach to implementing CI/CD in Android projects?
Answer:
- Use tools like GitHub Actions or Bitrise for automating build, testing, and deployment.
- Automate APK generation and upload to the Play Store.
- Integrate static code analysis tools like SonarQube.
Example in Practice:
In a food delivery app, CI/CD pipelines reduced release cycle times by 30%.
Note: Previously asked in Swiggy interviews.
🔟 How do you stay updated with the latest trends in Android development?
Answer:
- Follow the Android Developers Blog and Kotlin Updates.
- Join communities like Stack Overflow and Reddit’s r/androiddev.
- Attend industry events like DroidCon.
Example in Practice:
I learned Jetpack Compose by experimenting with it in personal projects after reading official documentation.
Note: Previously asked in LinkedIn interviews.
1️⃣1️⃣ – 2️⃣0️⃣ Additional Questions
Question | Company |
---|---|
11. Explain Data Binding and its benefits in Android. | Uber |
12. How do you handle app crashes in production? | Zomato |
13. What are deep links, and how do you implement them? | Airbnb |
14. How do you manage multiple API responses simultaneously? | Microsoft |
15. What are the challenges in Android development, and how do you solve them? | Spotify |
16. How do you optimize battery consumption in Android apps? | Samsung |
17. Difference between Serializable and Parcelable. | CRED |
18. How do you handle localization in Android? | Adobe |
19. How do you implement dark mode in an app? | Slack |
20. How do you secure API keys in Android? | Ola Cabs |
💡 Pro Tip: Interviewers love real-world examples. Make sure to share insights from your projects to highlight your hands-on expertise.
Share your interview question here in comments. We will publish without knowing your identity 🚀